Don't just instantiate a concrete class and use it straight away. You'll be regretting in the longer term Simple Factory Pattern
This is a continuation of the software design pattern series. You can checkout the previous posts from my-blog
Simple factory pattern
Ever been in a situation where when you need to change the declaration of a concrete class and you got to change in all the locations across your code base?Ever faced a situation where your client and concrete classes are coupled together so that when you try to introduce a similar concrete class and replace the old one, it gets harder?
Then you got to start programming to the interface/super-class instead of the implementation/sub-class. This can be achieved by introducing a factory for creating such implementations.
Ok. So how does it actually work?
Let's take an example of ordering a Pizza. The software takes type of the pizza as input and returns the respective pizza as output.Consider there are two types of pizzas.
- Pepperoni Pizza
- Cheese Pizza
Our typical code without factory pattern looks like this.
Now we could see that the orderPizza method is tightly coupled with the creation of concrete Pizza classes and whenever a new pizza type like PastaPizza gets introduced, the orderPizza method gets really messy as it's dealing directly with which concrete class in instantiated. It's probably time to encapsulate it.
Check the following code.
Now the complete responsibility of creation of the concrete Pizza classes are given to the PizzaFactory and it will be the single point of contact of instantiating those Pizza classes.
Cool. So give me a gist to implement Simple factory pattern?
In Simple factory pattern, just three main component gets involved excluding the Client.- Product Interface (Pizza)
- Concrete Product implementations (Cheese Pizza)
- Simple Factory implementation (SimplePizzaFactory)
-> ProductInterface
The interface which all the products the factory can create should be implementing.-> Concrete Product implementation
The Concrete classes implementing the Product interface. There are the classes which are needed to be instantiated from the factory.-> Simple Factory class
The main responsibility of this class is to get the args and depending on it, create the requested product and return it.Design Structure
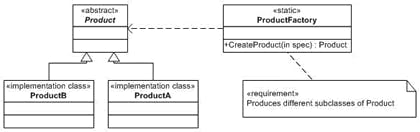
photo courtesy: link
In the coming posts, I will explain the variants of the factory patterns which will eliminate the limitations in the simple factory pattern.
Thank you. Hope this helps. Looking forward to more learning and sharing. Cheers :)
Connect, share and text me about your views. We can have some discussion :)